New Features For MSAL Python - Managed Identity and macOS Broker
Table of Contents
I’ve talked before about Microsoft Authentication Library (MSAL) for .NET on this blog, but I feel like I should bring attention to another product that our team ships that is massively adopted in the community - MSAL Python.
Adoption stats #
When I say massively, I am not exactly exaggerating. Here are the monthly downloads of the library, per version, as extracted from PyPI stats:
It’s growing quickly! More than 80 millions of downloads per month is nothing to sneeze at. We’re steadily approaching the two billion lifetime downloads mark (MSAL.NET hit one billion downloads just a few months back). And as the library grows, so is the demand for new capabilities that we’ve enabled across other languages - and not just in MSAL proper, but also in dependent libraries, such as the Azure Identity SDK, which uses MSAL under the hood.
There are two new features that I want to highlight - support for managed identity and support for macOS authentication brokers.
Managed identity #
Managed identities are really important as we move more and more workloads to the secretless paradigm. That is, what’s better than securing your secret keys to cloud resources? Not having secrets in the first place.
Starting with MSAL Python 1.29.0 (PyPI package is msal
), we introduced support for managed identities. Managed identities provide an automatically managed identity (comes from the name) in Microsoft Entra ID for services and APIs that they can use to talk to resources that support Microsoft Entra authentication. We support resources in Azure such as VMs, App Service, Azure Automation (Runbooks), Azure Functions, Service Fabric, and Azure Arc.
There are two types of managed identities - system assigned (a service principal is created and managed by the Azure resource, such as a virtual machine), or user-assigned (you manage the service principal separately from the Azure resource). MSAL Python supports both.
To acquire a token with a system-assigned managed identity, developers can use the newly-introduced ManagedIdentityClient
in tandem with SystemAssignedManagedIdentity
:
import msal
import requests
managed_identity = msal.SystemAssignedManagedIdentity()
global_app = msal.ManagedIdentityClient(
managed_identity, http_client=requests.Session())
result = global_app.acquire_token_for_client(
resource='https://vault.azure.net')
if "access_token" in result:
print("Token obtained!")
Using a user-assigned managed identity is, for all intents and purposes, following the exact same patter, but with a UserAssignedManagedIdentity
. Because you are managing the service principal directly, you will need to specify either client ID (client_id
), resource ID (resource_id
), or the object ID (object_id
):
import msal
import requests
managed_identity = msal.UserAssignedManagedIdentity(client_id='YOUR_CLIENT_ID')
global_app = msal.ManagedIdentityClient(
managed_identity, http_client=requests.Session())
result = global_app.acquire_token_for_client(
resource='https://vault.azure.net')
if "access_token" in result:
print("Token obtained!")
Our goal with the developer experience for managed identities is to make sure that it’s as intuitive and straightforward as possible - what you see above is what you can use in production.
One important thing to consider as you roll out applications that use managed identities through MSAL Python is caching. The managed identity implementation in the library supports in-memory caching out-of-the-box and you don’t need to do anything to enable it. It also supports cache extensibility, for scenarios where tokens need to be persisted. This is useful in a lot of special scenarios (such as CLI tool usage); however, we do not recommend sharing the managed identity token cache across multiple resources as this can result in unexpected access behaviors for identities in the cache (e.g., a token acquired for a node on one machine could be used on another node to gain access to resources said node should not have access to).
I recommend you check out our documentation to get a more in-depth overview of the integration.
macOS broker #
MSAL Python is a multi-workload library and is used not only on the backend but also on the frontend. For desktop applications that are using the library and are deployed on macOS devices we’ve introduced a new way to authenticate users, similar to how we’ve done it for Windows - through an authentication broker. This functionality is available starting with msal
1.31.0.
Using an authentication broker enables organizations to simplify how users authenticate with Microsoft Entra ID (that is - they can use Single Sign-On) from an application, as well as help them take advantage of future functionality that protects Microsoft Entra ID refresh tokens from exfiltration and misuse. Users get less sign-in prompts, and their tokens are protected from malicious actors who might want to take them somewhere else and access user data.
To use an authentication broker on macOS, a device needs to be managed by an organization (e.g., through Microsoft Intune) and use a supported broker application, such as Company Portal.
For developers, to make sure that their applications are compatible with brokered flows, they also need to leverage the broker-specific dependencies for msal
. Those can be installed with:
pip install msal[broker]>=1.31,<2
The code to use the broker is similar to what developers already use to authenticate users - it uses PublicClientApplication
, with a broker-specific argument:
from msal import PublicClientApplication
app = PublicClientApplication(
"CLIENT_ID",
authority="https://login.microsoftonline.com/common",
enable_broker_on_mac =True)
enable_broker_on_mac
ensures that when the application runs on a macOS device, it will first attempt to use the installed authentication broker (if any) before falling back to other alternatives, like the system browser. When the user is prompted for their credentials, they will see this:
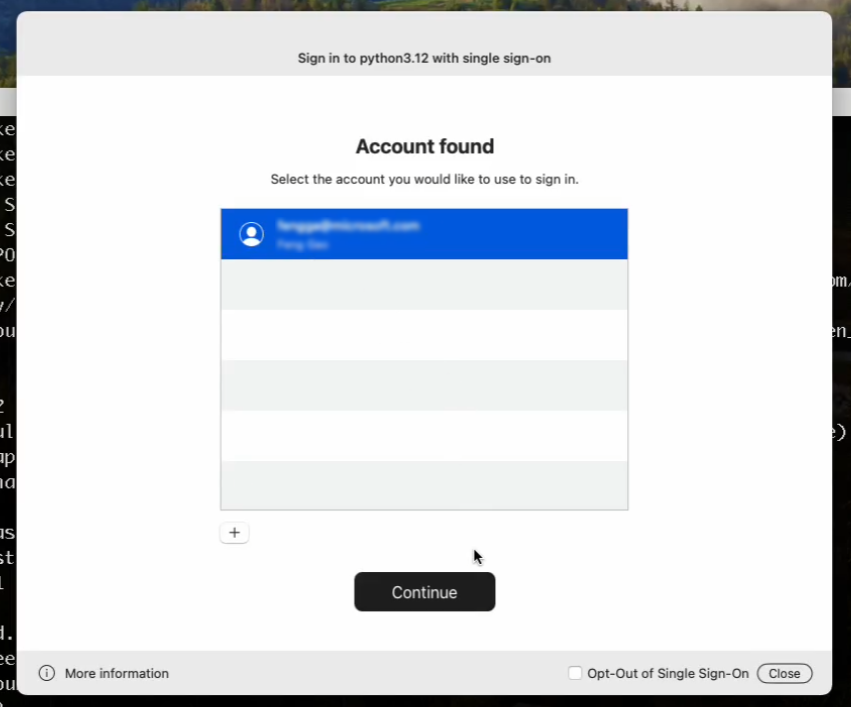
The enable_broker_on_mac
argument can also be used together with enable_broker_on_windows
for applications that are cross-platform and need broker-based authentication on Windows as well.
Lastly, to be able to use the broker, an application registration in Microsoft Entra ID needs to be properly configured to support the right redirect URIs. You can learn more about their format and requirements in our library documentation.
Feedback #
I wouldn’t be a product manager if I wouldn’t ask you for feedback at the end of this post. If you have any thoughts, comments, or suggestions around MSAL Python, do open an issue. We triage them regularly and are always looking forward to learning more about how we can make the developer experience better.